Payment gateway in the e-shop
The payment gateway allows display optimized for iframe. This functionality is suitable in case you do not want to redirect the payer to the payment gateway, but display it within your system. To display the payment gateway in an iframe, you need to use the "embedded" = true
parameter when creating the payment. The payment URL is generated in the standard way, but instead of redirecting the customer to the gateway, an iframe with the payment URL is displayed on the e-shop page.
There are two display options. Either you can display the payment gateway directly in the cart or using a pop-up window over your website. Implementing an iframe requires knowledge of web technologies. Iframe can only be used for card payment. For other payment methods, the payer will always be redirected to a new page.
If you are using the Content-Security-Policy
header on your website, it is necessary to allow all external contexts in iframes. We discuss this configuration in the Security section.
For the correct display of the payment gateway in an iframe on the web page, we recommend making the following modification to your web pages.
Setting the display of the payment gateway
HTML code
<div id="comgate-container">
<iframe id='comgate-iframe' allow="payment" src="[payment URL]" frameborder="0px"></iframe>
</div>
CSS styles
#comgate-container {
display: none;
position:absolute;
z-index: 9999;
left: 50%;
top: 30px;
overflow: auto;
margin-left: -250px;
}
#comgate-iframe {
width: 504px;
height: 679px;
}
@media (max-height: 700px) {
#comgate-iframe {
top: 0px;
}
}
JavaScript code
// function to open iframe with gateway
function comgateOpen() {
let comgate_container = document.getElementById("comgate-container");
comgate_container.style.display = "block";
}
// function to close iframe with gateway
function comgateClose() {
let comgate_container = document.getElementById("comgate-container");
comgate_container.style.display = "none";
}
To display the iframe, you need to call the comgateOpen()
function. For example, by binding to a user action (clicking a button, etc.). The comgateClose()
function then serves to possibly hide the iframe.
Example of calling the function to display the iframe by clicking the "Pay" button:
HTML code
<button id="comgate-open" onclick="comgateOpen()">Pay</button>
Redirecting the customer after completing the payment
After the payment is completed by the customer, they are redirected back to the e-shop on the URL that you have set in the client portal. We recommend performing one of the following modifications, which will ensure that the customer is redirected directly to the return URL and does not stay inside the iframe.
The first option is to redirect the external page to the URL you specified. This will refresh the entire page.
Javascript code for the internal page
window.top.location = window.self.location
The second option is to send a message from the page inside the iframe to the external page and process this event on the external page. This way, the entire page does not have to be refreshed.
Please note, the actual payment result needs to be obtained in the background in the usual way. For security reasons, it is not possible to rely on the result passed by a message from the iframe.
Javascript code for the internal page, which sends the external page a message with the payment ID and payment status, e.g., for a paid payment:
window.parent.postMessage('[payment id]|PAID', '*');
Javascript code for the external page, which processes the incoming message from the iframe:
// capture a message sent from the iframe using postMessage
if (window.addEventListener) {
window.addEventListener('message', function (e) {
// I get the id and payment result, the separator is a “pipe”
let message = e.data.split('|', 2);
let message_id = message[0];
let message_result = message[1];
let result = '';
if (message_result === 'PAID') {
// handling the PAID state
[...]
} else {
// handling other states, etc ...
[...]
}
}, false);
}
Payment gateway in the iframe from the payer's perspective
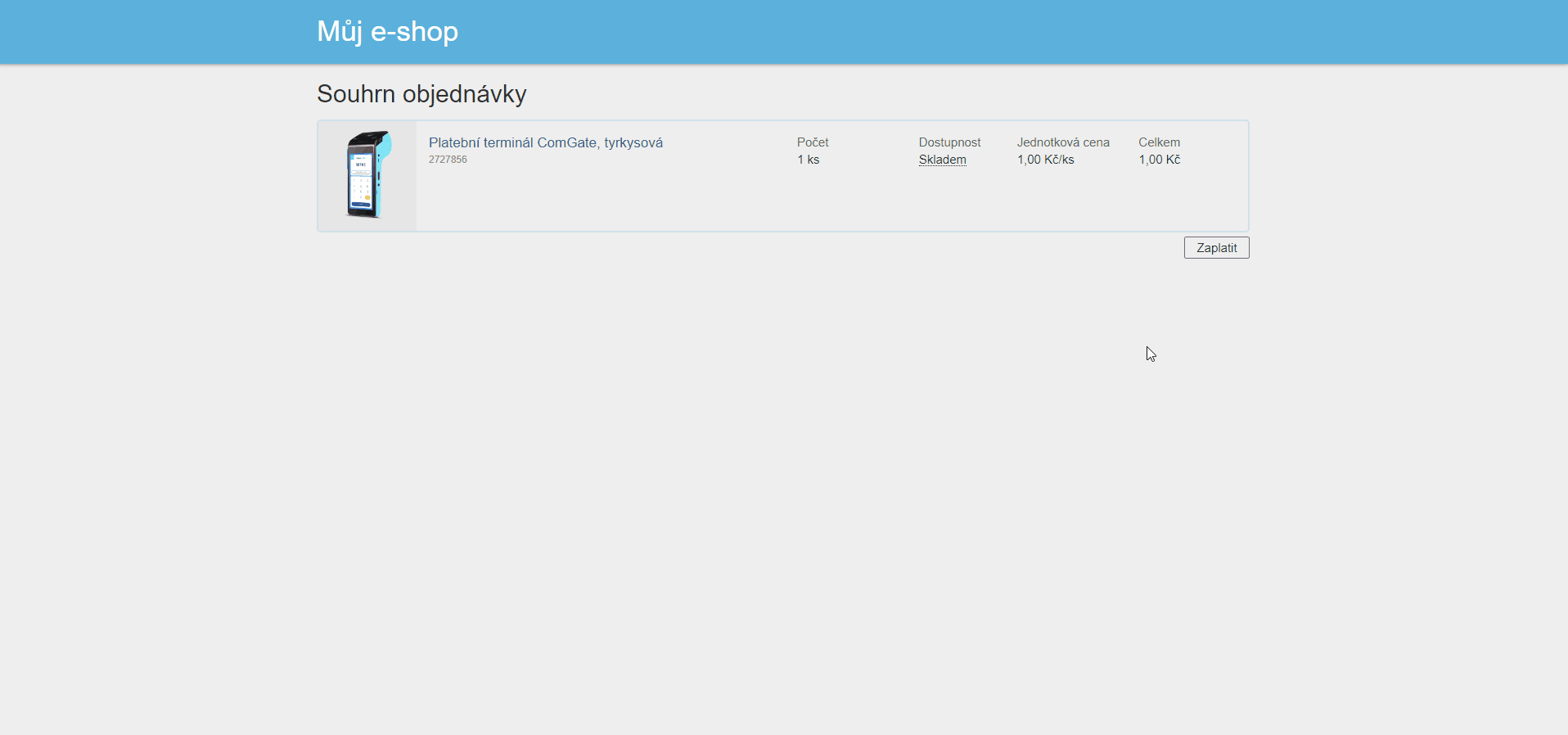
The Comgate payment gateway allows users to repeat the payment if they failed to complete the payment the first time. The display of the payment gateway in the iframe to the user depends on the chosen payment method and also whether the selection of payment methods is on the e-shop side or the Comgate side.
If the selection of payment methods is on the e-shop side and the payment is therefore created with a payment method already selected, the card payment method must be chosen to display the iframe. If the user failed to complete the payment the first time, only the card payment method is displayed again for the next attempt. This version is recommended.
If the e-shop does not create a payment with an already selected payment method, the user is shown a selection of payment methods in the iframe. Here, it is necessary to choose card payment. If the user failed to complete the payment, the selection of payment methods in the iframe is shown again for the next attempt.
If a method other than card payment is chosen at any step of the payment process, the payer is redirected to a new page. The user will not return to the iframe.
Template for displaying the gateway in the e-shop
For easy implementation of our payment gateway into your e-shop, you can use the available template implementations below.
HTML code
<!doctype html>
<html lang="cs">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Comgate :: working with iframe</title>
<style>
body,html {
background: #fff;
padding:0;
margin:0;
width: 100%;
height: 100%;
}
.page {
width: 100%;
background: #eee;
margin: 0 auto;
max-width: 800px;
}
.page .header h1 {
text-align: center;
margin: 0;
padding: 25px 15px;
font-size: 25px;
}
#comgate-iframe-box {
width: 450px; /* width is set automatically to 100% of screen width for small displays */
height: 700px; /* for the new gateway, you can dynamically adjust the height according to your needs */
margin: 0 auto;
}
#comgate-iframe-box .iframe {
width: 100%;
height: 100%;
}
@media (max-width: 450px) {
#comgate-iframe-box {
width: 100%;
}
}
</style>
</head>
<body>
<div class="page">
<div class="header">
<h1>Demonstration of working with iframe</h1>
</div>
<div id="comgate-iframe-box">
<!--
In iframe configuration:
use scrolling="off" for the new gateway
use scrolling="on" for the old gateway
Note: The URL address (src) of the established payment can change.
Always use the address returned by the API, and do not interfere with it.
-->
<iframe
class="iframe"
src="https://pay2.comgate.cz/init?id=XXXX-XXXX-XXXX"
allow="payment"
frameborder="0px"
scrolling="off">
</iframe>
</div>
</div>
</body>
</html>
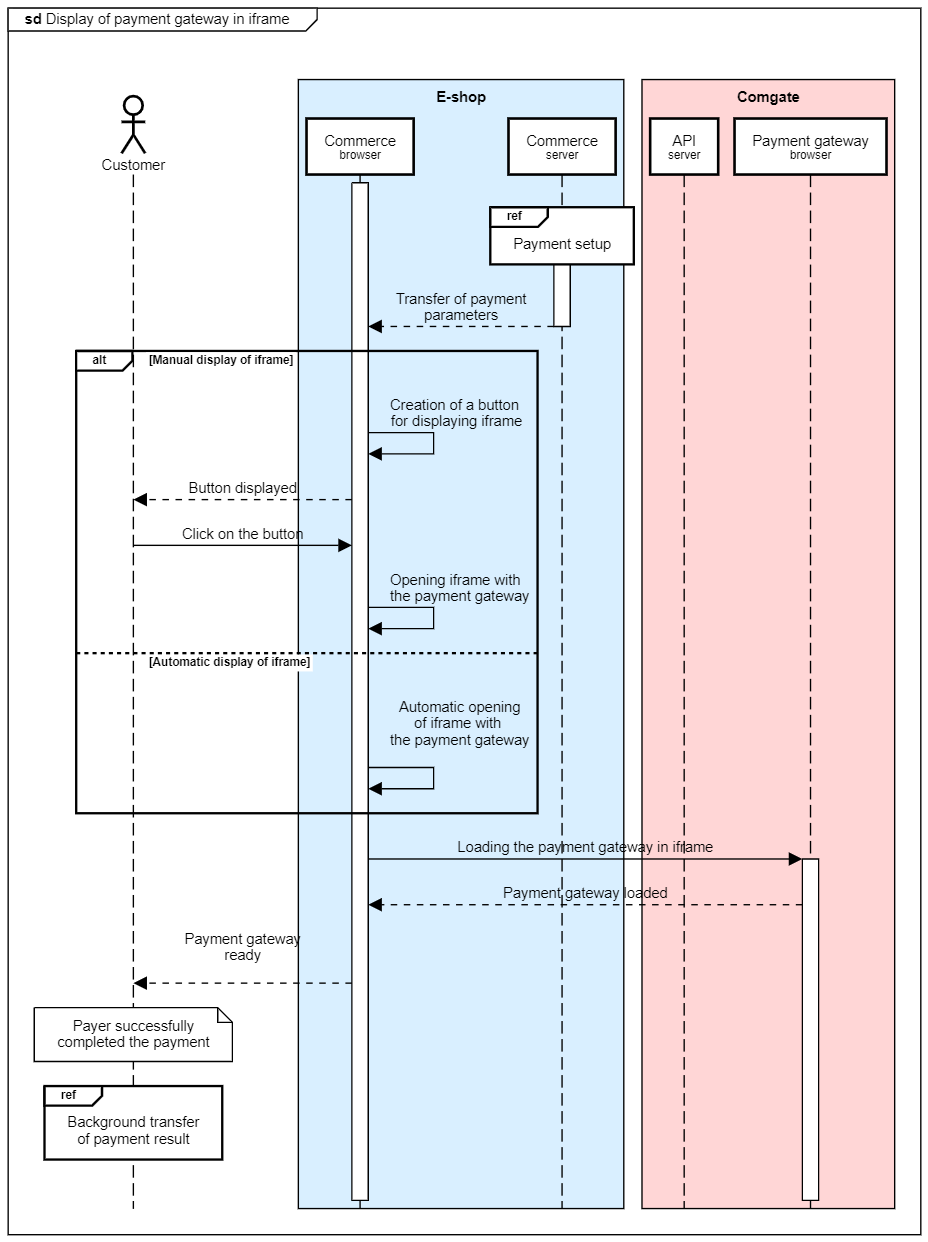